How to Create a Chat App: A Comprehensive Guide
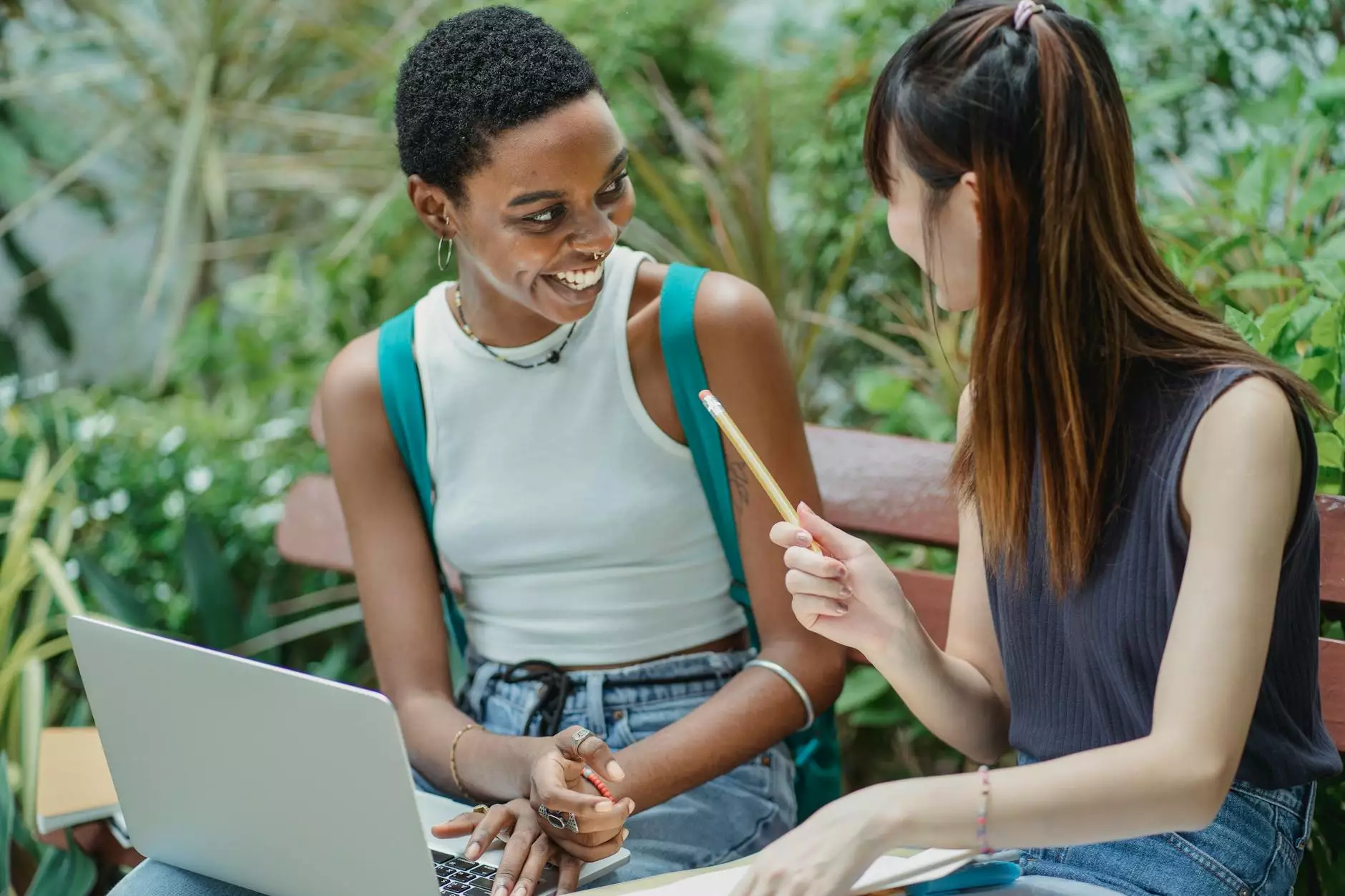
Introduction to Chat Applications
Chat applications have become an integral part of modern communication. They are used in various contexts, from casual chats among friends to professional communication in businesses. As technology continues to advance, the demand for feature-rich and user-friendly chat apps has skyrocketed. In this guide, we will explore the essential steps involved in how to create a chat app that stands out in today’s competitive market.
Understanding the Core Features of a Chat App
Before diving into the development process, it’s crucial to understand the core features that a successful chat app should offer. Here are the fundamental functionalities:
- User Authentication: Ensure secure sign-up and login mechanisms.
- Real-Time Messaging: Enable users to send and receive messages instantly.
- Group Chats: Allow multiple users to communicate in a single thread.
- File Sharing: Enable users to share images, documents, and other files.
- Push Notifications: Notify users of new messages even when the app is not open.
- Message History: Provide users with access to previous conversations.
- Emojis and Stickers: Enhance communication through expressive graphics.
- End-to-End Encryption: Ensure privacy and security of users’ messages.
Technology Stack for Developing a Chat App
Choosing the right technology stack is vital for the success of your chat application. Below is a recommended technology stack that incorporates both the front-end and back-end development:
- Front-End:
- React or Angular: For building an interactive user interface.
- HTML5 and CSS3: For structuring content and styling.
- Back-End:
- Node.js: Ideal for real-time applications due to its non-blocking nature.
- Express.js: A minimal and flexible Node.js web application framework.
- Socket.IO: For real-time bidirectional event-based communication.
- Database:
- MongoDB: A NoSQL database that stores data in JSON-like documents, suitable for chat applications.
- Cloud Services:
- AWS or Firebase: For hosting your application and databases.
- Twilio or Pusher: For simplifying messaging functionality.
Step-by-Step Guide on How to Create a Chat App
Now that we have the core features and technology stack defined, let’s go through a step-by-step process on how to create a chat app.
Step 1: Set Up Your Development Environment
Start by installing Node.js and your preferred code editor (like Visual Studio Code). Install necessary dependencies using npm (Node Package Manager) to set up your initial project.
Step 2: Initialize Your Project
Run the following command in your terminal to create a new Node.js project:
npm init -yThis command generates a package.json file to manage your project’s dependencies.
Step 3: Install Dependencies
Next, you need to install the required packages. Install Express, Socket.IO, and any other libraries you plan to use:
npm install express socket.io mongoose cors dotenvMongoose will be used for MongoDB object modeling, while CORS is essential for enabling your application to handle requests from different origins.
Step 4: Build the Server
Create a file named server.js. Set up an Express server and integrate Socket.IO:
const express = require('express'); const http = require('http'); const socketIo = require('socket.io'); const cors = require('cors'); const app = express(); const server = http.createServer(app); const io = socketIo(server); app.use(cors()); app.get('/', (req, res) => res.send('Chat Server Running')); io.on('connection', (socket) => { console.log('a user connected'); socket.on('chat message', (msg) => { io.emit('chat message', msg); }); socket.on('disconnect', () => { console.log('user disconnected'); }); }); server.listen(3000, () => { console.log('listening on *:3000'); });This code sets up a basic server that can handle WebSocket connections.
Step 5: Create the Client-Side Interface
Now, create an HTML file (index.html) for the front-end:
Chat AppStep 6: Implement Socket.IO on the Client
Now, add the client-side JavaScript in app.js:
const socket = io(); const form = document.getElementById('form'); const input = document.getElementById('input'); form.addEventListener('submit', function(e) { e.preventDefault(); if (input.value) { socket.emit('chat message', input.value); input.value = ''; } }); socket.on('chat message', function(msg) { const item = document.createElement('li'); item.textContent = msg; document.getElementById('messages').appendChild(item); window.scrollTo(0, document.body.scrollHeight); });Step 7: Style Your Chat App
Add CSS to enhance the user interface. Create a styles.css file:
body { font-family: Arial, sans-serif; margin: 0; padding: 20px; background-color: #f9f9f9; } ul { list-style-type: none; padding: 0; } li { padding: 8px; margin-bottom: 5px; background-color: #e0e0e0; border-radius: 5px; } form { display: flex; } input { flex: 1; padding: 10px; margin-right: 10px; } button { padding: 10px; }Step 8: Test Your Application
Run your server using the command:
node server.jsOpen multiple browser windows to test real-time messaging functionality.
Step 9: Deploy Your Chat App
Once you’re satisfied with your application, deploy it using platforms like Heroku, AWS, or DigitalOcean. Ensure that your database is set up in the cloud as well.
Best Practices for Chat App Development
As you embark on your journey of how to create a chat app, consider the following best practices:
- Optimize for Performance: Ensure your app is fast and responsive. Consider implementing lazy loading and optimizing media sizes.
- Focus on User Experience: A seamless user experience is critical. Pay attention to UI/UX design principles.
- Implement Security Measures: Use HTTPS, sanitize inputs, and enforce authentication to protect user data.
- Scalability: Design your app to accommodate growth and handle increased traffic efficiently.
- Regular Updates: Keep your app updated with the latest features and security fixes.
Conclusion
Creating a chat app can be a rewarding venture, whether for personal use, business communication, or community interaction. By following the steps outlined in this guide, you are now equipped with the knowledge of how to create a chat app that is not only functional but also user-friendly and secure. Engage with your users, gather feedback, and continuously improve your application to meet their needs. Happy coding!
© 2023 nandbox.com - All rights reserved.